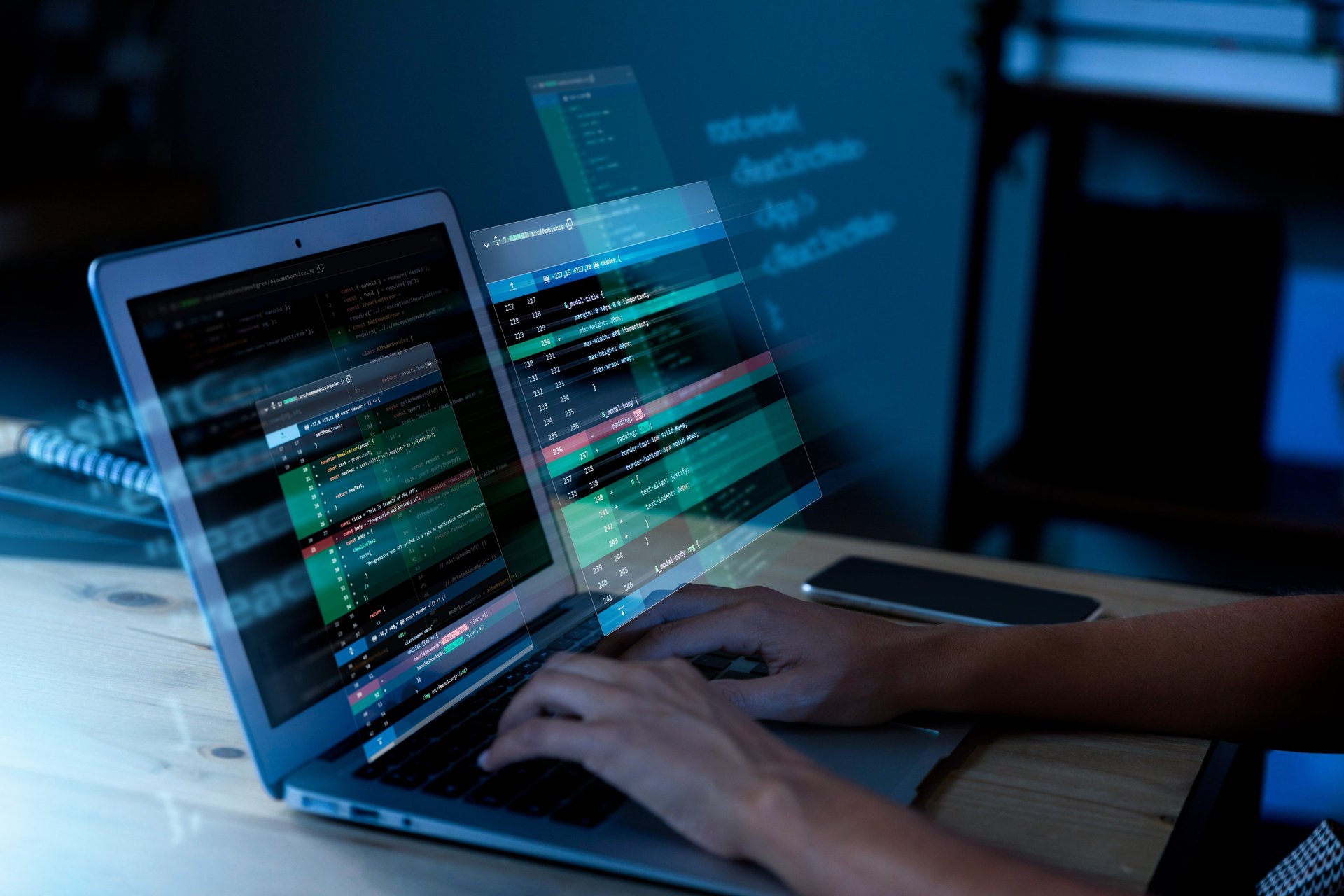
Selenium with Java
UI Test Automation is a process of automating the user interface testing of an application. Selenium with Java, Maven, and TestNG are popular tools used for UI test automation. Selenium is an open-source tool that supports various programming languages, including Java. Maven is a build automation tool that helps in managing dependencies and building projects. TestNG is a testing framework that supports multiple test types, including unit, functional, and integration testing. By using these tools, UI test automation becomes more efficient and reliable, resulting in faster test execution and better quality software. With the combination of Selenium, Java, Maven, and TestNG, developers and testers can automate their UI tests and ensure that their applications are functioning as intended.
Selenium with Java training is for professionals who wants to upgrade their career to Automation and wants to become expert in Selenium. The course will help you to learn concepts about Core Java, Selenium WebDriver, TestNG, and Maven. The course covers multiple automation frameworks with hands on exercises on projects. The training will be delivered in the form virtual classes. The course is designed from scratch where it covers the basics and advanced concepts of Java as well.
Audience
Professionals who wants to groom their career in automation using Selenium
Novice who wants to learn automation and get selected in an IT company
Professionals who wants to switch their jobs by learning new skill.
USP of the course
The course is curated by industry experts with 20+ years of experience who are having extensive experience in Automation with different tools and are aware of real time issues.
Course covering Core Java as well as Selenium
Challenging Problem statements and Solutions
The trainers are handpicked from the companies and has extensive experience in real time automation challenges.
Assessment at regular intervals.
Interview Tips and Questions for preparation.
Selenium Course Content
The topics that we cover in Selenium/Java/TestNG/Maven training are as follows:
Automation Concepts
What is Automation
Automation Characteristics
Different tools in the market for Automation
Introduction to Java
Installing Java
Installing Eclipse
First Eclipse Project
First Java program
Concept of class file
Datatypes in Java
String class
If statements
Loops, Arrays and Functions
Conditional and concatenation operators
While, For Loops
Practical Examples with loops
Usage of loops in Selenium
Single Dimensional, Two Dimensional Arrays
Practical usage of arrays in Selenium
What are Functions?
Function Input Parameters
Function Return Types
OOPS Concepts
Local Variables
Global Variables
Meaning of static
Why is main method static?
Static and Non-Static Variables
Static and Non-Static Functions
Creating Objects in Java
Object and Object References
Call by reference and Value
Constructors
Usage of Objects in Selenium
Concept of Inheritance
Interface
Overloading and Overriding Functions
Example on inheritance
Object Class
Usage of Inheritance in Selenium
Packages/Access Modifiers/Exception handling
Relevance of Packages
Creating Packages
Accessing Classes Across Packages
Accessing modifiers - Public, Private, Default, Protected
Exception Handling
Exception handing with try catch block
Exception and Error
Throwable Class
Final and Finally
Throw and Throws
Different Types of Exceptions
Need of exception handling in Selenium framework
Collection API
Introduction to Collections API
ArrayList Class
HashTable Class
Using ArrayList and HashTable in Selenium framework
String, File Handling, Log4j, /Handling XLS files
String class and functions
Reading Properties File in Java
Concept of jar file
POI API in java
Reading/Writing Microsoft XLS Files
Log4j API for Logging
Usage of Log4J in Selenium
Selenium Web driver
Download Maven dependencies and integrate with Maven project in eclipse
Architecture of selenium webdriver 3
Drivers for Firefox, IE, chrome
Geckodriver for Firefox in Selenium 3
First Selenium Code
Close and Quit difference
Disabling notifications, maximizing, Disabling infobars
Page Load Strategy
WebDriver Interface
WebElement Interface
Working with chrome and IE
WebDriver DesiredCapabilities Class
Proxy settings with webdriver-3/Working with proxy Servers
HTMLUnit driver and desired capabilities
Working with different browsers without changing code
Managing https Certificate Errors
Various locator strategies
XPATHS
HTML language tags and attributes
Identifying WebElements using id, name, class
Finding Xpaths to identify objects
Absolute and relative Xpaths
Can 2 elements have same xpath
Can 1 element have multiple xpaths
Creating customized Xpaths
Element with variable Ids - startswith, contains, endswith
Finding xpaths in different browsers - Mozilla, Chrome and IE
Handling Dynamic objects/ids on the page
Implicit Wait
NoSuchElementException and InvalidSelectorException
Managing Input fields, Buttons
Managing/Identifying Links with xpaths
Extracting More than one object from a page
Extracting all links of a page/Bulk extraction of objects
Hidden components
isDisplayed function
Extracting Objects from a specific area of a web page
StaleElementReferenceException
Broken Links Validation
Finding whether object is present on page or not
Handling drop down list
Select Class in Selenium API
Auto Suggestive drop down handling
Managing radio buttons and Checkboxes
Taking Screenshots of the web pages
How to Google out errors-Self sufficiency
Finding Coordinates of a Web Object
Actions class in Webdriver
Handling CSS menu with Action class
Unpredictable Popup
Selecting date from Calendar
Building test cases for framework
Page Object Model With Page Factory
Concept of Page object model
Brief discussion about goals to be achieved in Page Object Model design
Concept of inheritance and encapsulation in java
Concept of Page Object Model
Where can Page Object module be used
Issues faced in developing page object model
Build a maven Project
Build Page classes
Implement PageFactory Design Pattern
Annotations in Page Object Model Page factory
Complete the flow
Build the base Page class
Build reusable validation functions
Encapsulate common features of pages
Build the base test class and common functions
Remove Hardcoding/Use Constants file
Implementing reporting-Extent reports
Logging and page classes
Take screenshots and put them in reports
Read data from xls
Reporting failure and putting screenshots of failure in reports
Creating custom utility functions for data reading
Complete test cases
Batch Running test cases using Maven
Selenium Grid
Implement GRID - Parallel execution on multiple VMs
Cloud platform integration
Execute script on cloud platforms e.g. BrowserStack
Cross Browser testing using BrowserStack
Continuous Integration/ Continuous Deployment
Understanding of Azure Devops
Creating Pipelines on Azure Devops for continuous Testing
Understanding Jenkins
Scheduling Execution on Jenkins